Introduction
React has become a cornerstone of modern web development, empowering developers to build dynamic and interactive user interfaces. At the heart of React’s magic are two key concepts: state and props. These might sound like abstract jargon to beginners, but understanding them is your ticket to mastering React. We will learn What are React State and Props. The beginners guide step by step.
React state and props are the bedrock of communication within components they dictate how data flows and how components behave. But the journey to understanding these concepts is not just technical it’s a fascinating experience filled with curiosity, “aha” moments, and eventually, confidence. Let’s embark on this journey together and unpack the beauty of state and props in the simplest, most human way.
What Are React State and Props?
Before diving deep, it’s essential to define these concepts in the simplest terms.
- State: Think of the state as the memory of a component. It’s where a component stores data that can change over time. The state is local and specific to the component it resides in.
- Props (short for properties): Props are like the settings you pass to a component. They allow components to receive data and behave in a way that’s controlled from the outside.
The difference? If state is about what a component knows, props are about what a component is told.
State: The Heart of Dynamic Components
What Is State?
In the context of React, state refers to the data or variables that are managed within a component. Think of state as the dynamic memory of a React component it allows the component to keep track of changing data and re-render the user interface accordingly. Without state, a React application would be static and unresponsive to user interactions.
State is what makes React components truly dynamic, enabling features like updating form inputs, toggling elements, or even fetching and displaying data from APIs. It transforms components from passive structures into active participants in building interactive and engaging user experiences.
Characteristics of State
- Local to the Component: State belongs to the specific component where it is defined. It’s private and cannot be directly accessed by other components.
- Mutable: Unlike props, which are read-only, state can be changed or updated using the proper methods.
- Triggers Re-renders: When the state of a component changes, React automatically re-renders the component to reflect the updated state in the UI.
- Initialized in Components: In class components, state is initialized in the constructor. In functional components, state is managed using the
useState
Hook. - Temporary Storage: State holds data temporarily, typically for as long as the component is mounted.
Example Scenario: Role of State
Imagine you’re creating a shopping cart in a React application. The items a user selects for their cart could be stored as part of the state. As the user adds or removes items, the state updates, and React re-renders the cart to display the updated list of products.
Managing State in React
- In Class Components, state is managed using the
this.state
object. To update state, you use thesetState
method. - In Functional Components, state is handled using React Hooks, specifically the
useState
Hook. Hooks provide a simpler and more intuitive way to manage state.
Props: The Lifeline for Component Communication
What Are Props?
In React, props (short for properties) are a way to pass data from one component to another, specifically from a parent component to a child component. Props allow components to be dynamic and reusable, making React applications efficient and scalable. They are a foundational concept in React, and understanding how they work is essential for developing well-structured and maintainable applications.
Characteristics of Props
- Immutable: Props cannot be changed or modified by the receiving component. They are read-only and used to display or utilize the data passed by the parent component.
- External Data Source: Props are received by a component from its parent, making them external to the component. This is the opposite of state, which is internal to a component.
- Facilitates Communication: Props act as a communication channel between parent and child components, allowing data to flow seamlessly in a React application.
- Reusable Components: By passing different props to the same component, you can customize its behavior or appearance, enhancing reusability.
Props remind me of the gentle guidance from a mentor. They don’t impose; instead, they provide information that allows components to shine in their unique roles.
Why Are Props Important?
Props are the lifeline of React’s component-based architecture. They enable you to create a hierarchy of components, with data flowing down from parent to child components. This allows React applications to be modular, making it easier to manage complex UI structures.
For example, a button component could receive props to customize its label and style. By using props, you avoid duplicating code and ensure that your components are flexible and adaptable to various needs.
When to Use Props?
Props are ideal for:
- Passing data from parent to child components
- Configuring reusable components (e.g., buttons, cards, headers)
- Displaying static content that doesn’t change within the child component
How Props Enhance React Applications
Imagine a scenario where you’re designing a user profile card component. Props allow you to pass specific information like the user’s name, image, and bio from the parent component to the child. This makes your component adaptable for different users without duplicating the code. This versatility is what makes props an indispensable tool in React development.
State vs. Props: How They Compare
Feature | State | Props |
---|---|---|
Definition | Data managed internally | Data passed externally |
Mutability | Mutable (can be updated) | Immutable |
Usage | For dynamic behavior | For static configuration |
Ownership | Belongs to the component | Belongs to parent components |
These distinctions might seem subtle at first, but understanding the divide is like discovering the difference between left brain and right brain thinking—they complement each other beautifully.
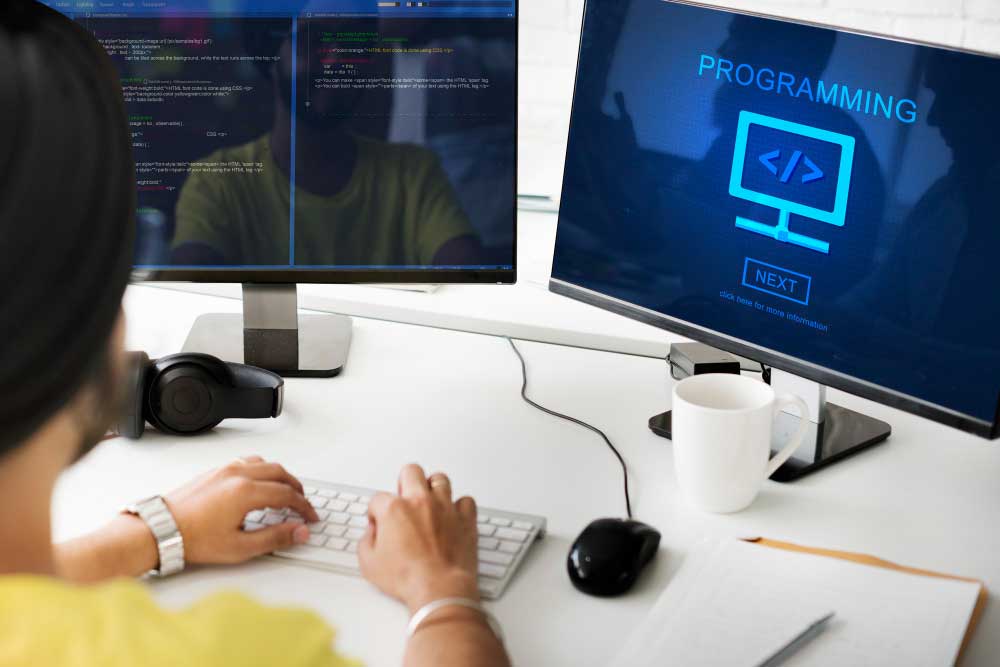
FAQs
1. Can a component have both state and props?
Absolutely! Components often use both state and props to function efficiently. State handles internal data, while props manage external configuration.
2. Can props change during the component’s lifecycle? Props are immutable from the perspective of the child component. However, they can be updated by the parent component, which re-renders the child with new data.
3. What happens when state is updated? Updating the state triggers a re-render of the component, ensuring the UI stays in sync with the data.
4. Are state and props specific to React? While they are central to React, similar concepts exist in other frameworks. However, React’s implementation is uniquely elegant.
5. Can I use state in functional components? Yes! With the introduction of Hooks in React 16.8, functional components can manage state using the useState
Hook.
Case Studies
- Building a Counter App: A student built their first React app: a counter. They used state to store the count and props to display it in a child component. The project demystified these concepts and laid a solid foundation for their learning.
- Dynamic Theming: A startup implemented a dynamic theme toggler using state to manage the current theme (light/dark) and props to pass the theme data to styled components.
- Form Handling: A team managing a complex form used state to track user inputs and props to validate data before submission. The combination resulted in an intuitive user experience.
- E-commerce Product Listings: An online store utilized state to manage product availability and props to display product details in a grid layout, achieving a seamless shopping experience.
- Real-time Chat Application: A chat application used state to store message history and props to pass user-specific settings, creating a personalized experience for each user.
Personal Insights
- The “Lightbulb Moment” When I first understood state, it felt like I’d unlocked a superpower. Suddenly, components weren’t just blocks of code—they were alive, responsive, and purposeful.
- Embracing Props Props taught me the value of collaboration—not just in coding but in life. Just as props empower components to work together, teamwork enriches everything we do.
- Overcoming Fear of Hooks Transitioning from class components to functional ones was daunting. But once I grasped Hooks, state management felt intuitive. It was a lesson in embracing change.
- Balancing State and Props Finding the balance between state and props is like learning to juggle. It’s tricky at first, but with practice, it becomes second nature.
- The Human Element Understanding state and props made me realize that even in coding, the human element matters. Whether it’s sharing data (props) or managing emotions (state), React mirrors how we interact as people.
Official Resources and External Links
- React Docs on State and Lifecycle
- React Props Overview
- Introducing React Hooks
- React FAQ: Component Communication
- Managing State in React
Conclusion
State and props are the lifeblood of React components. They may seem intimidating at first, but as you explore their nuances, you’ll discover how intuitive and powerful they are. Remember, learning React is not just a technical journey it’s an emotional one. Every challenge you face and every concept you grasp adds to your growth as a developer.
React is more than just a library. It’s a philosophy that encourages us to think in components, embrace reusability, and create seamless user experiences. So take a deep breath, dive into state and props, and let your curiosity lead the way. You’ve got this!
Understanding React Components: Functional vs. Class Components
3 thoughts on “What are React State and Props? The Beginners Guide!”